TestKthMaxElement.cxx File Reference
Include dependency graph for TestKthMaxElement.cxx:
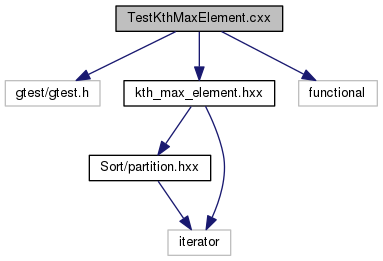
Go to the source code of this file.
Functions | |
TEST (TestSearch, MaxKthElement) | |
TEST (TestSearch, MinKthElement) | |
Function Documentation
TEST | ( | TestSearch | , |
MaxKthElement | |||
) |
Definition at line 41 of file TestKthMaxElement.cxx.
52 EXPECT_EQ(ksortedArray.begin() + 4, MaxKthElement<IT>(ksortedArray.begin(), ksortedArray.end(), 4));
55 EXPECT_EQ(ksortedArray.begin(), MaxKthElement<IT>(ksortedArray.begin(), ksortedArray.begin(), 0));
58 EXPECT_EQ(ksortedArray.begin(), MaxKthElement<IT>(ksortedArray.begin(), ksortedArray.begin() + 1, 0));
IT MaxKthElement(const IT &begin, const IT &end, unsigned int k)
Definition: kth_max_element.hxx:50
TEST | ( | TestSearch | , |
MinKthElement | |||
) |
Definition at line 76 of file TestKthMaxElement.cxx.